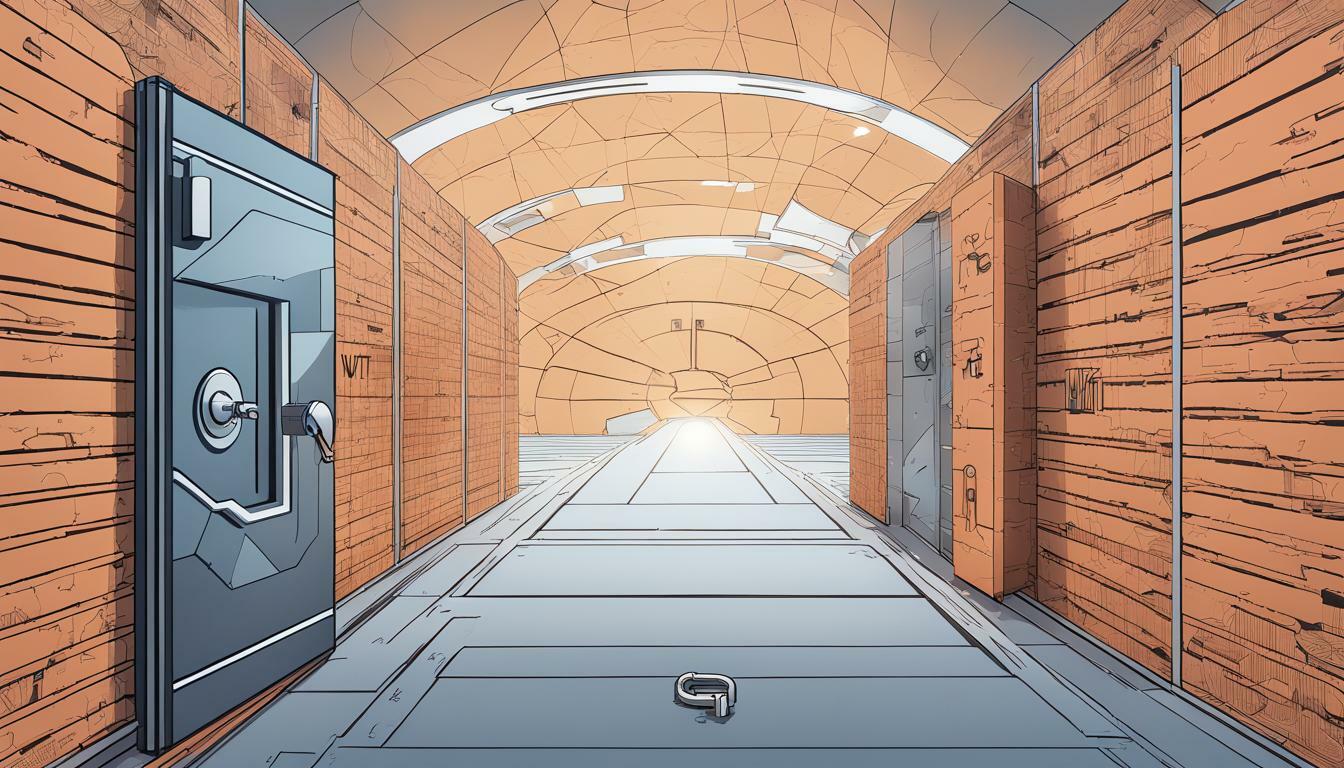
Welcome to our guide on securing your API with JSON Web Token (JWT) authentication in .NET Core. As data breaches become increasingly common, it’s crucial to protect your API and the sensitive data it handles. Implementing JWT authentication can significantly enhance the security and reliability of your API, and .NET Core provides a powerful authentication framework for achieving this.
In this article, we’ll guide you through the process of implementing JWT authentication in your .NET Core application. We’ll cover a range of topics, including API security, JWT implementation, and best practices for authentication in .NET Core. By the end of this guide, you’ll have a solid understanding of how to secure your API with JWT authentication.
Key Takeaways:
- Implementing JWT authentication can significantly enhance the security and reliability of your API.
- .NET Core provides a powerful authentication framework for achieving this.
- API security is crucial to protect sensitive data from data breaches.
- JWT implementation involves three components: header, payload, and signature.
- Best practices for JWT authentication include token validation, payload design, and secure token transmission.
Understanding API Security
API security is crucial in today’s technology-driven world. An API, or application programming interface, is like a digital bouncer that controls access to your application. It acts as an intermediary between your application and the outside world, allowing authorized users to access your data and services while blocking unauthorized access. Therefore, securing your API is paramount to the overall security of your application.
One of the most common security threats to web APIs is unauthorized access. A malicious user can gain access to your API by bypassing authentication measures and perform actions that can cause significant damage to your application and data. Additionally, other common threats include injection attacks, denial of service attacks, and broken authentication and session management.
By implementing strong authentication mechanisms like JWT, you can add an additional layer of security to your web API. JSON Web Token (JWT) is a self-contained, compact, and secure way to transmit information between parties as a JSON object. It is a standard for token-based authentication and authorization and is widely used by many companies and developers.
Introduction to JWT Authentication
JSON Web Tokens (JWTs) are a popular method for implementing authentication in web APIs. A JWT consists of three parts: a header, a payload, and a signature. The header and payload are base64-encoded JSON strings, and the signature is used to validate the authenticity of the token.
When a user logs in, the server generates a JWT containing relevant user information and returns it to the client. The client then includes the JWT in the headers of subsequent requests to the API. The server can then validate the JWT and grant access to authorized resources.
One of the benefits of JWT authentication is that it allows for stateless authentication, meaning the server doesn’t have to keep track of session data. As long as the JWT is valid, the server can trust that the user is authenticated and authorized.
Setting Up Authentication in .NET Core
Implementing JWT authentication in .NET Core is a straightforward process that involves configuring your application to use the JSON Web Token middleware. With just a few lines of code, you can enable API authentication to enhance the security of your application.
The first step in setting up authentication in .NET Core is to add the necessary NuGet packages to your project. You will need to install the Microsoft.AspNetCore.Authentication.JwtBearer package, which provides the JWT bearer authentication middleware, as well as the System.IdentityModel.Tokens.Jwt package, which contains the JWT token handler.
Next, you need to add the JWT authentication middleware to your application pipeline. This can be done by calling the AddAuthentication method in the ConfigureServices method of your Startup.cs file and specifying the default authentication scheme:
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = Configuration["Jwt:Issuer"],
ValidAudience = Configuration["Jwt:Audience"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:SecretKey"]))
};
});
Here, we are registering the JWT bearer authentication middleware and defining the token validation parameters. You will need to provide the secret key, issuer, and audience values in your appsettings.json or environment variables.
Once you have added the JWT authentication middleware, you can apply the [Authorize] attribute to your API controllers or actions to require authentication for access:
[ApiController] [Authorize] [Route("api/[controller]")] public class ProductsController : ControllerBase
{
//...
}
In this example, we are applying the [Authorize] attribute at the controller level to require authentication for all actions in the ProductsController.
Finally, you need to configure the authentication middleware in the Configure method of your Startup.cs file by calling the UseAuthentication method:
app.UseAuthentication();
With these configurations in place, your .NET Core application is now set up to use JWT authentication for API security.
Protecting Your API Routes
Once you have implemented JWT authentication in your .NET Core application, it’s important to protect your API routes from unauthorized access. By applying appropriate authorization policies, you can ensure that only authenticated users can access your API resources.
To protect your API routes, you first need to define the necessary authorization policies. This can be done in the ConfigureServices method of your Startup class, as shown below:
Note: This code is an example and should be adapted to your specific application needs.
services.AddAuthorization(options => { options.AddPolicy("ApiUser", policy => policy.RequireClaim("scope", "api.access")); });
In this example, we define a policy named “ApiUser” that requires the “api.access” claim to be present in the JWT. This claim can be added to the JWT payload during authentication.
Once you have defined the necessary policies, you can apply them to your API routes using the [Authorize] attribute:
Note: This code is an example and should be adapted to your specific application needs.
[Authorize("ApiUser")] [HttpGet] public IActionResult Get() { // API logic here }
By adding the [Authorize(“ApiUser”)] attribute to your API routes, you ensure that only authenticated users with the “api.access” claim can access these routes.
If an unauthorized user attempts to access a protected API route, your application will return a 401 Unauthorized response. You can customize this response by defining an IAuthorizationFilter.
In addition to protecting your API routes, it’s important to handle unauthorized requests and provide appropriate error responses. You can do this by implementing an IExceptionHandler and registering it in the Configure method of your Startup class.
By following these best practices for protecting your API routes, you can enhance the security of your application and ensure that only authorized users can access sensitive data.
Handling Token Expiration and Refresh
Implementing a token refresh mechanism is crucial for ensuring uninterrupted access to your API. In this section, we will discuss the process of handling token expiration and refreshing tokens in .NET Core.
Token Expiration
When a user logs in to your application, they receive a JSON Web Token (JWT) that contains their authentication credentials. This token has an expiration time, after which it becomes invalid, and the user must log in again to receive a new token.
To prevent unauthorized access to your API, it is essential to set a reasonable token expiration time. You should consider various factors while setting the token expiration time, such as your application’s security needs and the user experience. A common practice is to set the token expiration time to a few hours to a day, depending on the needs of your application.
Token Refresh
When the token expires, the user must log in again to access the API. However, this can be a cumbersome process for the user, especially if they are in the middle of a task. To avoid this interruption, you can implement a token refresh mechanism.
The token refresh mechanism is a process where the user sends a refresh token to the server, and the server returns a new JWT without requiring the user to provide their credentials again. This process ensures that the user experiences uninterrupted access to your API.
Implementing Token Refresh
To implement the token refresh mechanism, you must create a new API endpoint that receives the user’s refresh token and returns a new JWT if the refresh token is valid. You can set a different expiration time for the refresh token, depending on your application’s needs.
When a user requests an API endpoint with an expired JWT, you can return a specific error code to indicate that the token has expired. The client can then use the refresh token to request a new JWT, which they can use to access the API again.
It is essential to ensure that the refresh token is securely stored and transmitted to prevent unauthorized access to your API. You can use various techniques such as encrypting the refresh token, storing it in a secure database, or using HTTPS to transmit the refresh token securely.
Revoking Tokens
In some scenarios, such as when a user logs out or changes their password, you may need to revoke their tokens to prevent unauthorized access to your API. To revoke a token, you can add the token to a blacklist or an invalid token store. When a user requests an API endpoint with a revoked token, you can return an error code indicating that the token is invalid.
Tip: | Use a unique identifier, such as a GUID, to identify each token to simplify token revocation. |
---|
Implementing token refresh and revocation mechanisms can significantly enhance the security of your API and the user experience. By following the best practices outlined in this section, you can ensure that your API remains secure, even if a user’s token is compromised.
Implementing JWT Authentication Best Practices
Implementing JSON Web Token (JWT) authentication in your .NET Core application is a powerful security measure to protect your API from unauthorized access. However, to ensure the maximum level of security, it is essential to follow some best practices.
1. Validate Tokens Properly
One of the most critical best practices in JWT authentication is to validate tokens correctly. Always ensure that the token is valid, signed by a trusted authority, and has not expired. Additionally, validate the token’s signature to ensure that it has not been tampered with.
2. Design the Token Payload Carefully
The payload of a JWT contains essential information about the user and the application. Therefore, it is crucial to design the payload carefully. The payload should contain only the necessary information and avoid including sensitive data that could compromise the application’s security.
3. Securely Transmit Tokens
When transmitting tokens, it is essential to ensure that they are transmitted securely. Always use HTTPS to encrypt the token and avoid transmitting the token in the URL or using query parameters. Additionally, consider using cookies to transmit the token instead of the header.
4. Use Refresh Tokens
Implementing a token refresh mechanism is one of the best practices to ensure uninterrupted access to your API. Refresh tokens allow users to obtain a new access token without requiring them to log in again. This approach enhances the user experience while maintaining the security of your API.
5. Revoke Tokens Securely
Token revocation is necessary when a user’s access needs to be revoked due to a security breach or a user leaving the organization. When revoking tokens, ensure that the process is secure. Consider implementing a blacklist or a database to store revoked tokens.
By following these best practices, you can enhance the security of your API and protect your application’s sensitive data.
Conclusion
In conclusion, implementing JWT authentication is a crucial step in securing your API in .NET Core. By understanding the importance of API security and the benefits of using JWT authentication, you can enhance the security of your application. Setting up authentication in .NET Core involves configuring the necessary code snippets and securely storing JWT secrets. Additionally, protecting your API routes using authorization policies restricts access to specific routes based on a valid JWT. Handling token expiration and refresh ensures uninterrupted access to your API, while following best practices, such as token validation and securing token transmission, further strengthens security.
By following the steps outlined in this article, you can successfully implement JWT authentication in your .NET Core application. As a result, you can protect sensitive data and strengthen the security of your application. Remember to keep up-to-date with any new developments in the world of API security and adjust your security measures accordingly.
FAQ
Q: What is API security?
A: API security refers to the measures taken to protect an API (Application Programming Interface) from unauthorized access, attacks, and data breaches. It involves implementing authentication mechanisms, encryption, and authorization policies to ensure the confidentiality, integrity, and availability of the API and the data it handles.
Q: Why is API security important?
A: API security is crucial because APIs often handle sensitive data and serve as gateways to valuable resources. Without proper security measures, APIs can be vulnerable to unauthorized access, data breaches, and attacks such as injection, cross-site scripting (XSS), and denial of service (DoS). Securing APIs helps protect user and system data, maintains trust with consumers, and prevents financial losses and reputational damage.
Q: What is JWT authentication?
A: JWT (JSON Web Token) authentication is a method of user authentication and authorization commonly used in web APIs. It involves issuing and validating tokens, which are digitally signed and contain claims about the user and their permissions. JWT authentication is stateless, meaning it does not require server-side storage of session data, making it scalable and suitable for distributed systems.
Q: How does JWT authentication work?
A: JWT authentication works by generating and validating JSON Web Tokens. When a user successfully logs in, the server generates a JWT containing the user’s claims and signs it using a secret key. The JWT is then sent to the client, who includes it in subsequent requests. The server verifies the integrity and authenticity of the token’s signature and extracts the user’s claims to determine their identity and permissions.
Q: How do I set up JWT authentication in .NET Core?
A: To set up JWT authentication in .NET Core, you need to configure the authentication middleware in your application’s startup code. This involves specifying the authentication scheme, validating the incoming JWT, and setting the appropriate authorization policies. You also need to store and manage the secret key securely for signing and verifying the tokens. Detailed code snippets and instructions can be found in the relevant section of this article.
Q: How can I protect my API routes using JWT authentication?
A: To protect your API routes using JWT authentication, you can apply authorization policies that require the presence of a valid JWT with the necessary claims. By decorating your controllers or individual action methods with the `[Authorize]` attribute, you can ensure that only authenticated users with the correct permissions can access those routes. Unauthorized requests can be handled by returning appropriate error responses.
Q: What should I do when a JWT token expires?
A: When a JWT token expires, you should implement a token refresh mechanism. This involves generating a new token using a refresh token or by exchanging an expired token for a new one with a renewed expiration time. The refresh token is typically stored securely on the client side and can be used to obtain a new access token without requiring the user to log in again. Proper handling of expired tokens helps maintain uninterrupted access to your API.
Q: What are some best practices for implementing JWT authentication?
A: Some best practices for implementing JWT authentication include carefully validating tokens for integrity and authenticity, properly designing the token payload to minimize sensitive information exposure, and securing token transmission using HTTPS. It is also recommended to enforce strong security measures for storing and managing the secret key and to periodically rotate the key for added security. Adhering to these best practices can enhance the security and reliability of your API authentication.